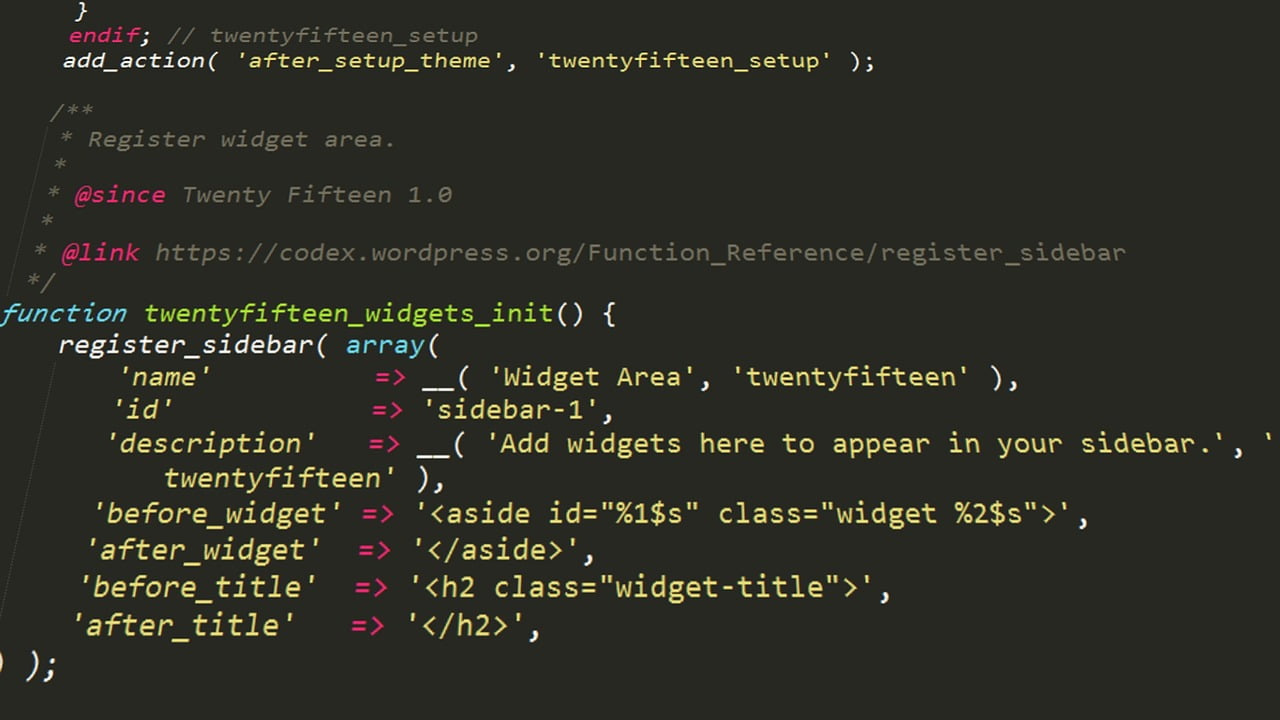
WordPress has 100’s of functions that can be used on daily basis while building any type of website in WordPress. Here are some of those functions :
1. get_theme_mod() :
It is used for theme modification and to retrieve all the themes modification.
Syntax:
get_theme_mod(string $name, string|false $default = false)
Example:
function my_theme_customize_register( $wp_customize ) {
$wp_customize->add_setting( 'header_bg_color', array( //This is a header
background color
'default' => '#ffffff',
'transport' => 'refresh',
) );
$wp_customize->add_control( new WP_Customize_Color_Control(
$wp_customize, 'header_bg_color', array(
'label' => __( 'Header Background Color', 'my_theme' ),
'section' => 'colors',
'settings' => 'header_bg_color',
) ) );
}
add_action( 'customize_register', 'my_theme_customize_register' );
2. apply_filters() :
This function can be used to create a new filter hook by simply
calling this function with the name of the new hook specified using
the $tag parameter.
Syntax: apply_filters(string $tag, mixed $value)
Example: // The filter callback function.
function example_callback( $string, $arg1, $arg2 ) {
// (maybe) modify $string.
return $string;
}
add_filter( 'example_filter', 'example_callback', 10, 3 );
/*
Apply the filters by calling the 'example_callback()' function
that's hooked onto `example_filter` above.
'example_filter' is the filter hook.
'filter me' is the value being filtered.
$arg1 and $arg2 are the additional arguments passed to the
callback.*/
$value = apply_filters( 'example_filter', 'filter me', $arg1, $arg2 );
3. add_filter() & add_action() :
add_filter() and add_action() are available before any plugin is loaded. So we can use
both in the first line of your plugin or theme.
syntax: add_filter( string $tag, callable $function_to_add, int
$priority = 10, int $accepted_args = 1)
Example: function example_callback( $example ) {
// Maybe modify $example in some way.
return $example;
}
add_filter( 'example_filter', 'example_callback' );
4. get_option() :
We can't directly access an array element with the function, it just doesn't support it.
get_option function is used to retrieve a value from from options table based on option name.
Syntax: get_option( string $option, mixed $default = false )
Example: <?php get_option( 'My name is Prateek Umrao', "I don't have anything
written. " ); ?>
5. esc_url() :
To use the esc_url() function, pass the URL you want to clean as an argument.
Syntax: esc_url( string $url, array $protocols = null, string $_context =
‘display’ )
Example: $url = "http;//example.com/link?var='some&";
echo esc_url( $url ); //> http://example.com/link?var=&#039some&#038
$url='data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAABIAAAD///////neHiwAAA
AF3RST/+8RwZ==';
echo esc_url( $url ); //> ''
var_dump( esc_url( '' ) ); // string(0) ""
var_dump( esc_url( false ) ); // string(0) ""
var_dump( esc_url( null ) ); // string(0) ""
6. absint() :
This function is convert all the value into abslute value.
Syntax: absint( $maybeint );
Example: echo absint( 'number' ); // 0
echo absint( 0 ); // 0
echo absint( 10 ); // 10
echo absint( -10 ); // 10
echo absint( '-10' ); // 10
echo absint( 10.5 ); // 10
echo absint( '10.5' ); // 10
echo absint( 'str' ); // 0
echo absint( 'str-10' ); // 0
echo absint( '-10str' ); // 10
echo absint( '10str' ); // 10
echo absint( true ); // 1
echo absint( false ); // 0
echo absint( array('12') ); // 1
echo absint( array() ); // 0
7. get_template_part() :
Basically, get_template_part() allows theme developers to set up an order of specificity of
template files. Think of it similarly to specificity as it applies to CSS selectors.
Syntax: get_template_part( string $slug, string $name = null, array $args = null )
Example: get_template_part( 'template-parts/featured-image', null,
array(
'class' => 'featured-home',
'data' => array(
'size' => 'large',
'is-active' => true,
)
)
8. get_post_type() :
Returns the theme's post templates for a given post type. Retrieves the permalink for an
attachment. Checks for changed slugs for published post objects and save the old slug. Sets
categories for a post.
Syntax: get_post_type( int|WP_Post|null $post = null ): string|false
9. get_the_ID() :
This function returns the ID of the current post in the WordPress loop or returns false if the
$post variable is not set.
Syntax: $id = get_the_ID();
10. the_content() :
The the_content() displays the content of a certain page or post. You know that pages and
posts are of course editable by users. The the_content() function gets the outputted HTML and
places it on the right place in a template file
Example: <?php if ( have_posts() ) : while ( have_posts() ) : the_post();
the_content();
endwhile; else: ?>
<p>Sorry, no posts matched your criteria.</p>
<?php endif; ?>
use: Basically, get_template_part() allows theme developers to set up an order of specificity of
template files. Think of it similarly to specificity as it applies to CSS selectors.
syntax: get_template_part( string $slug, string $name = null, array $args = null )
Example: get_template_part( 'template-parts/featured-image', null,
array(
'class' => 'featured-home',
'data' => array(
'size' => 'large',
'is-active' => true,
)
)